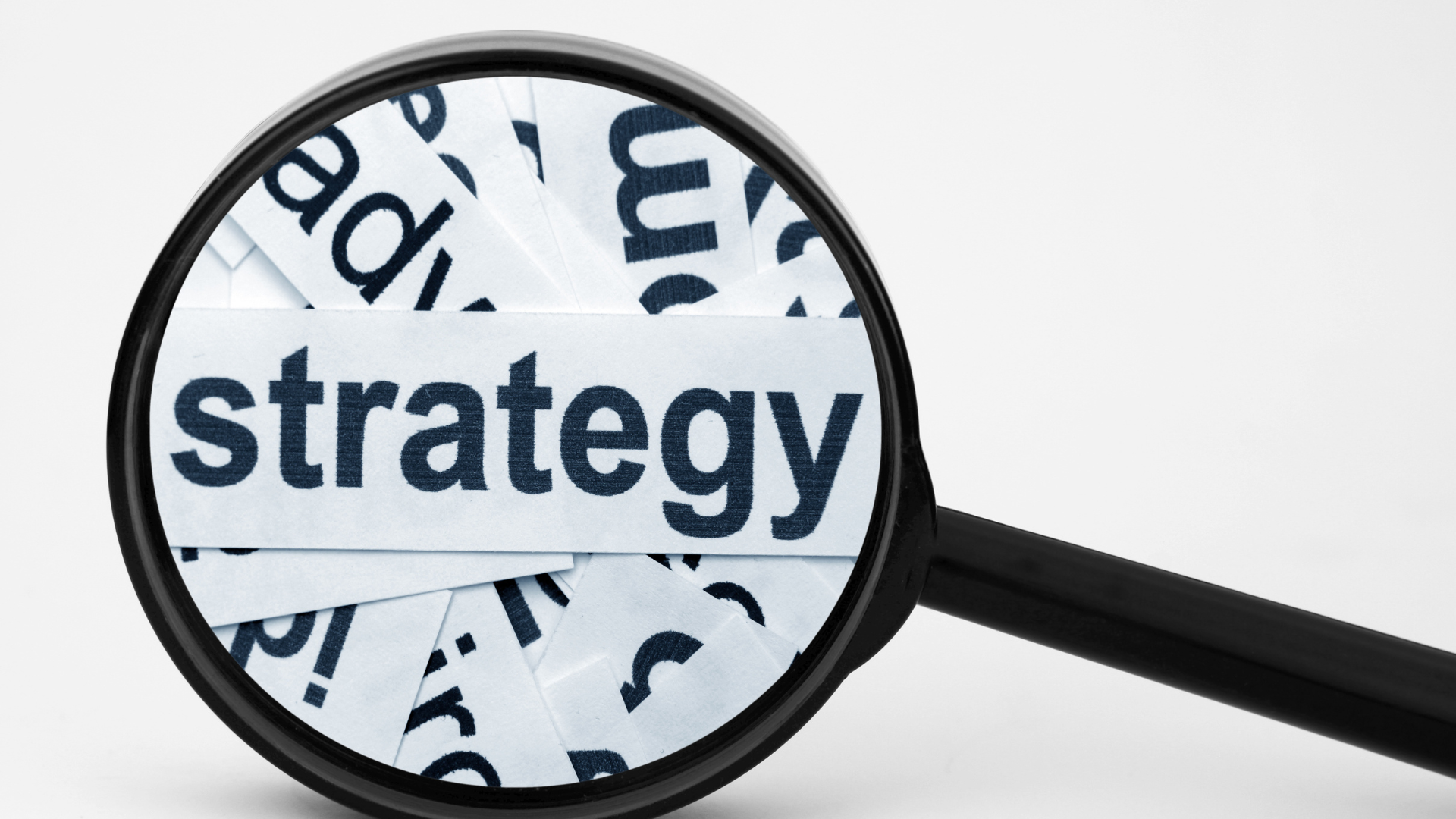
Intelligent Test Execution and Prioritization with Gen AI for Effective Test Strategies
_______ Sankar SanthanaramanHaving the right kind of test suites is just not enough as more often than not, the testing teams work on a time pressure, requiring them to prioritize the tests within the available time. This blog post delves into how Gen AI is revolutionizing test execution and prioritization, enabling testing teams to optimize their processes, focus on high-risk areas, and strike the perfect balance between coverage and efficiency.
Using Gen AI to Optimize Test Execution Order
Optimizing the order of test execution is crucial for early bug detection and efficient use of testing resources. Gen AI brings a new level of sophistication to this process:
1. Historical Data Analysis
Gen AI models can analyze vast amounts of historical test data to identify patterns in test failures and successes. This analysis helps in predicting which tests are more likely to fail in the current build.
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Assume we have historical data
X = np.array([test_features]) # Features of tests (e.g., complexity, last failure time)
y = np.array([test_results]) # Historical results (0 for pass, 1 for fail)
# Split data and train model
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Predict failure probability for new tests
new_tests = np.array([new_test_features])
failure_probabilities = model.predict_proba(new_tests)[:, 1]
# Sort tests by failure probability
sorted_test_indices = np.argsort(failure_probabilities)[::-1]
2. Dependency-Aware Scheduling
Gen AI can understand complex dependencies between tests and create an execution order that respects these dependencies while optimizing for early defect detection.
def topological_sort(graph):
visited = set()
stack = []
def dfs(node):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(neighbor)
stack.append(node)
for node in graph:
if node not in visited:
dfs(node)
return stack[::-1]
# Example usage
test_dependencies = {
'test1': ['test2', 'test3'],
'test2': ['test4'],
'test3': [],
'test4': []
}
execution_order = topological_sort(test_dependencies)
3. Dynamic Reordering
Gen AI models can dynamically adjust the test execution order based on real-time results, prioritizing tests that are more likely to uncover issues in problematic areas.
4. Resource Optimization
By considering factors such as test duration, resource requirements, and potential impact, Gen AI can create an execution order that maximizes the utilization of available testing resources.
Predicting High-Risk Areas for Focused Testing
Identifying high-risk areas allows testing teams to allocate resources more effectively. Gen AI excels at this task through:
1. Code Change Analysis
Gen AI can analyze code changes to predict which areas of the application are most likely to be affected and potentially introduce bugs.
from difflib import unified_diff
def analyze_code_changes(old_code, new_code):
diff = list(unified_diff(old_code.splitlines(), new_code.splitlines()))
changed_functions = set()
for line in diff:
if line.startswith('+ def ') or line.startswith('- def '):
function_name = line.split('def ')[1].split('(')[0]
changed_functions.add(function_name)
return changed_functions
# Example usage
old_code = """
def func1():
pass
def func2():
return True
"""
new_code = """
def func1():
return False
def func2():
return True
def func3():
pass
"""
changed_functions = analyze_code_changes(old_code, new_code)
print("Changed functions:", changed_functions)
2. Defect Prediction
By analyzing historical defect data, code complexity metrics, and developer information, Gen AI can predict which parts of the codebase are most likely to contain defects.
3. User Behavior Analysis
Gen AI can analyze user behavior patterns to identify critical paths and frequently used features, ensuring these areas receive thorough testing.
4. Complexity Assessment
Gen AI can evaluate the complexity of different modules or functions to identify areas that are inherently more prone to errors.
import ast
def calculate_cyclomatic_complexity(func_ast):
complexity = 1
for node in ast.walk(func_ast):
if isinstance(node, (ast.If, ast.While, ast.For, ast.And, ast.Or)):
complexity += 1
return complexity
def analyze_code_complexity(code):
tree = ast.parse(code)
complexities = {}
for node in ast.walk(tree):
if isinstance(node, ast.FunctionDef):
complexity = calculate_cyclomatic_complexity(node)
complexities[node.name] = complexity
return complexities
Example usage
code = """
def simple_function():
return True
def complex_function(x):
if x > 0:
for i in range(x):
if i % 2 == 0:
print(i)
else:
while x < 0:
x += 1
return x
"""
complexities = analyze_code_complexity(code)
print("Function complexities:", complexities)
Balancing Coverage and Efficiency in Test Execution
Achieving comprehensive test coverage while maintaining efficiency is a delicate balance. Gen AI helps strike this balance through:
1. Intelligent Test Case Selection
Gen AI can select a subset of test cases that provide maximum coverage with minimum redundancy, based on code coverage analysis and historical test results.
2. Risk-Based Testing
By assigning risk scores to different parts of the application, Gen AI can create a testing strategy that allocates more resources to high-risk areas while still ensuring baseline coverage for lower-risk components.
def risk_based_test_selection(tests, risk_scores, coverage_threshold):
selected_tests = []
current_coverage = 0
Sort tests by risk score in descending order
sorted_tests = sorted(tests, key=lambda t: risk_scores[t], reverse=True)
for test in sorted_tests:
if current_coverage < coverage_threshold:
selected_tests.append(test)
current_coverage += test.coverage
else:
break
return selected_tests
Example usage
class Test:
def __init__(self, name, coverage):
self.name = name
self.coverage = coverage
tests = [Test("test1", 0.2), Test("test2", 0.3), Test("test3", 0.15), Test("test4", 0.25)]
risk_scores = {"test1": 0.8, "test2": 0.6, "test3": 0.9, "test4": 0.5}
coverage_threshold = 0.7
selected = risk_based_test_selection(tests, risk_scores, coverage_threshold)
print("Selected tests:", [test.name for test in selected])
3. Adaptive Testing Strategies
Gen AI can dynamically adjust the testing strategy based on real-time results, focusing more on areas where issues are being discovered while reducing effort on stable components.
4. Predictive Resource Allocation
By predicting the time and resources required for different tests, Gen AI can create execution plans that maximize coverage within given time and resource constraints.
def optimize_test_execution(tests, available_time, available_resources):
Sort tests by value (e.g., risk score / execution time)
sorted_tests = sorted(tests, key=lambda t: t.value_score, reverse=True)
selected_tests = []
total_time = 0
total_resources = 0
for test in sorted_tests:
if total_time + test.execution_time <= available_time and \
total_resources + test.required_resources <= available_resources:
selected_tests.append(test)
total_time += test.execution_time
total_resources += test.required_resources
return selected_tests
Example usage
class Test:
def __init__(self, name, execution_time, required_resources, value_score):
self.name = name
self.execution_time = execution_time
self.required_resources = required_resources
self.value_score = value_score
tests = [
Test("test1", 10, 2, 0.8),
Test("test2", 15, 3, 0.7),
Test("test3", 5, 1, 0.9),
Test("test4", 20, 4, 0.6)
]
available_time = 30
available_resources = 5
optimized_tests = optimize_test_execution(tests, available_time,
available_resources)
print("Optimized test execution:", [test.name for test in optimized_tests])
Conclusion
Generative AI is transforming test execution and prioritization, enabling testing teams to work smarter, not harder. By optimizing test execution order, predicting high-risk areas, and balancing coverage with efficiency, Gen AI helps organizations achieve higher quality software with fewer resources.
However, it's crucial to remember that while Gen AI provides powerful insights and optimizations, human expertise remains invaluable. The most effective testing strategies combine the analytical power of AI with the domain knowledge and intuition of experienced testers.
As Gen AI continues to evolve, we can expect even more sophisticated approaches to test execution and prioritization. From more accurate risk predictions to real-time adaptive testing strategies, the future of AI in software testing is bright. By embracing these technologies and best practices, testing teams can significantly enhance the effectiveness and efficiency of their testing processes, ultimately leading to higher quality software delivered more rapidly to end-users.